PHP, originally created by Danish-Canadian programmer Rasmus Lerdorf in 1994, quickly emerged as a cornerstone of web development. Initially conceived as a simple set of CGI(Common Gateway Interface) binaries written in C, PHP (Personal Home Page, later rebranded as “Hypertext Preprocessor”) evolved into a powerful server-side scripting language.
PHP is a recursive acronym for Hypertext Preprocessor. This means that it refers to itself. The first “P” stands for PHP, and the second “P” stands for Preprocessor. The Hypertext part refers to the fact that PHP is often used to generate HTML pages that can be displayed in a web browser.
It is an open-source server-side scripting language used for web development and enables developers to create dynamic and interactive web pages. One interesting feature of PHP is its ability to generate PDF documents.
Generating PDF documents with PHP can be extremely useful in various scenarios, such as creating invoices, reports, or even e-books. It allows developers to create visually appealing documents that can be easily printed, shared, and stored.
PDF documents generated with PHP can also contain interactive elements such as hyperlinks, form fields, and multimedia content.
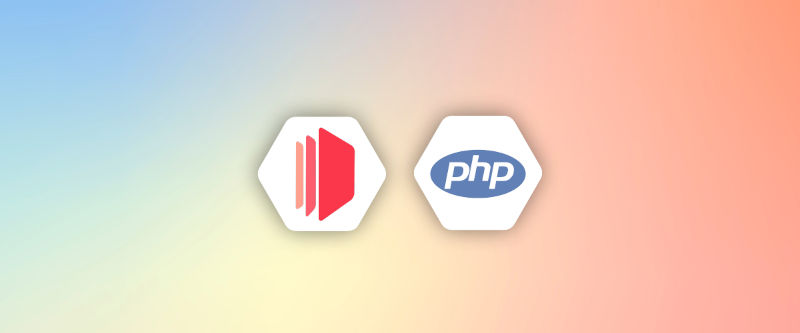
There are various libraries available to generate PDF documents with PHP. The following are the four popular PHP libraries to help you to generate PDFs:
- FPDF
- TCPDF
- DOMPDF and
- wkhtmltopdf
These libraries provide a wide range of features for creating PDF documents, such as setting page size, margins, fonts, and images.
In this article, I will briefly discuss some of these popular PHP libraries for PDF generation along with some code snippets and show you how to generate a PDF document using PHP.
In the second part of the article, we will also introduce a cloud-based PDF generation API – CraftMyPDF.
1. Common libraries to generate PDFs in PHP
PHP is an incredibly versatile language that has many benefits for web developers. One of the most impressive features of PHP is its ability to effortlessly generate PDF documents. With the help of powerful libraries, converting your files to PDF has never been easier.
Here are just a few of the top libraries that can assist you in creating beautiful, dynamic PDF documents:
1.1 FPDF – Lightweight PDF Generation
FPDF is a PHP library that allows you to create PDF documents smoothly. Even if you’re a complete newbie to PHP and PDF generation, you can quickly get started with this powerful library.
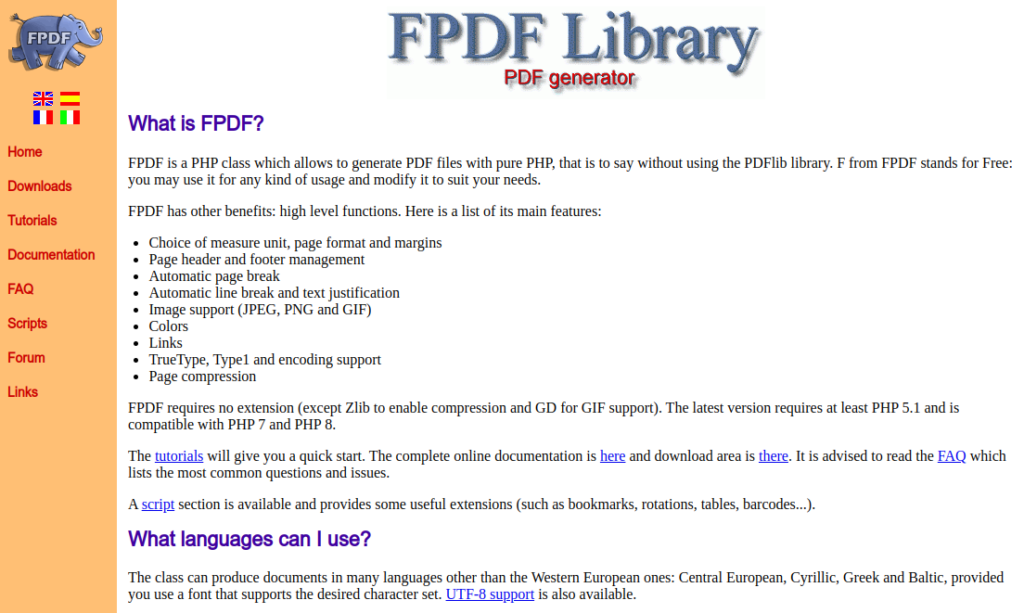
Its straightforward API and minimalistic approach make it easy to integrate into PHP applications, allowing developers to focus on content generation rather than complex configurations. FPDF’s lightweight nature ensures fast rendering times, making it a popular choice for projects where simplicity and performance are key priorities.
Here’s what you need to do:
i. Download the FPDF library from its official website.
ii. Once you’ve downloaded it, you can extract the files and save them in a newly created directory/folder called fpdf. You can name your directory however you like.
iii. Next, create a new PHP file called “newFPDFScript” (or any name you like) in the same directory you just created.
iv. Include the FPDF library in your newly created PHP script file using the “require” command.
<?php
require('./fpdf.php');
?>
v. Create a new instance of the FPDF class.
<?php
require('./fpdf.php');
class mypdf extends FPDF{
}
$pdf = new mypdf();
?>
vi. Once you’ve created your class, you can start adding content to your PDF document. For example, you can add a new page using the “customizePage” function:
<?php
require('./fpdf.php');
class mypdf extends FPDF{
function customizePage() {
$this->SetFont('Arial', 'B',16);
$this->Cell(40,10,'Welcome to CraftMyPDF!');
}
}
$pdf = new mypdf();
// Call the AddPage method to add a page to the PDF document
$pdf->AddPage();
$pdf->customizePage();
$pdf->Output();
?>
Save the file and test on your localhost. Your localhost URL should look similar to this http://localhost/fpdf/newFPDFScript.php
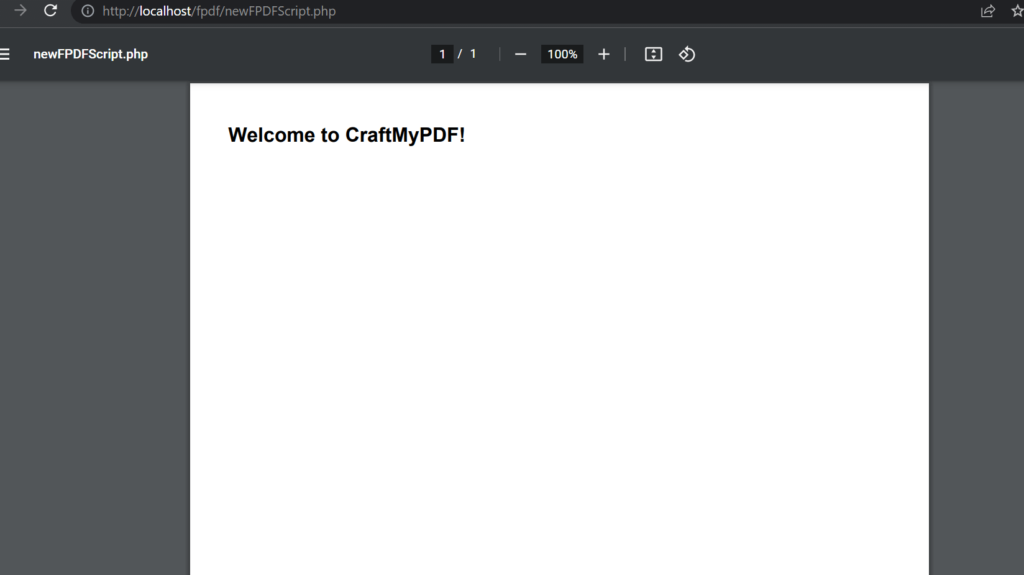
In this example, we created a new instance of the FPDF class and called the customizePage method to customize a page in our PDF document.
We then set the font and font size using the SetFont method, and added some text to the PDF document using the Cell method.
Finally, we called the AddPage method to add a page to the PDF document and output the PDF document using the Output method.
1.2 DOMPDF – Using the DOM method
Dompdf is a PHP library that allows you to convert HTML documents to PDF format. It is open source and is based on the PHP DOM extension. It uses the CSS Parser to convert CSS styles into inline style attributes and also supports a variety of HTML features, including tables, images, and basic HTML and CSS layouts.
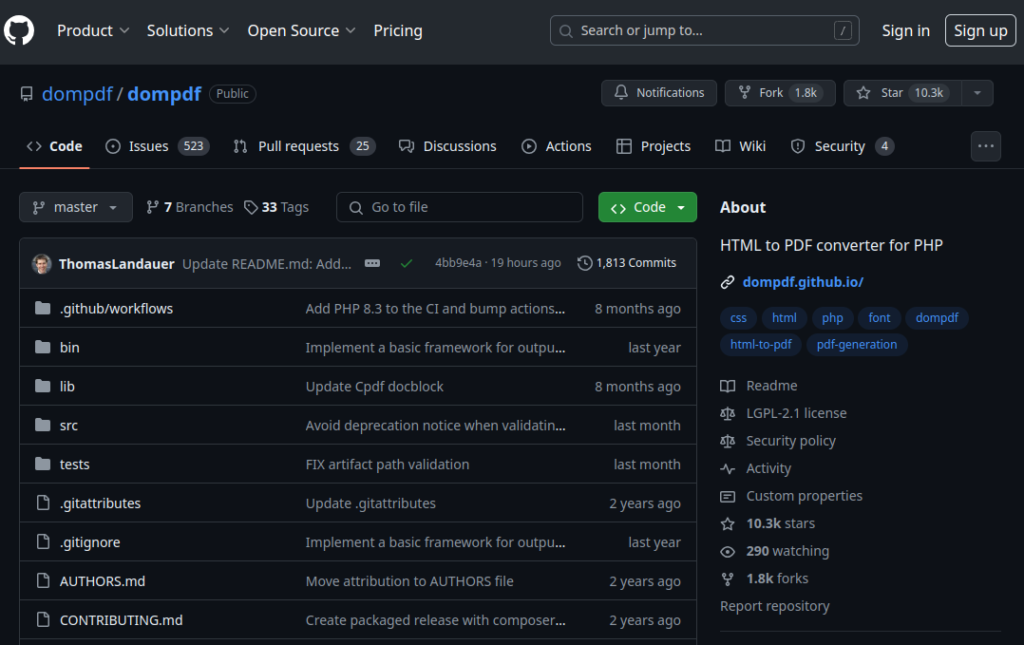
The library is designed to be easy to use and integrate into your PHP application. You can create a PDF document by simply passing an HTML string to the Dompdf library, which will generate a PDF file that you can then save to your server or send to the client.
Dompdf also supports advanced features like custom fonts, page numbering, headers, and footers. It also has built-in support for Unicode and right-to-left languages. The library can be installed via composer using the following command
composer require dompdf/dompdf
Alternatively, you have the option of cloning the repository from Github onto your system. Once you have cloned the repository, navigate to the folder you cloned and create a new file named “index.php”. Then, insert the following code snippet into the file.
<?php
// Include autoloader
//Make sure you link the file correctly using your own localhost url
require_once 'C:\xampp\htdocs\dompdf\autoload.inc.php';
// Reference the Dompdf namespace
use Dompdf\Dompdf;
// Instantiate and use the dompdf class
$dompdf = new Dompdf();
$html='
<style>
table {
font-family: arial;
width:400px;
border-collapse: collapse;
}
td, th {
border: 1px solid black;
text-align: left;
padding: 8px;
}
tr:nth-child(even) {
background-color: seagreen;
}
</style>
<h1>Welcome to CraftMyPDF</h1>
<h3>List of Registered users </h3>
<table>
<tr>
<th>Email</th>
<th>Username</th>
</tr>
<tr>
<td>[email protected]</td>
<td>vicboy</td>
</tr>
<tr>
<td>[email protected]</td>
<td>forever_janet</td>
</tr>
</table>
';
// Load HTML content
$dompdf->loadHtml($html);
// (Optional) Setup the paper size and orientation
$dompdf->setPaper('A4', 'landscape');
// Render the HTML as PDF
$dompdf->render();
// Output the generated PDF to Browser
$dompdf->stream();
?>
Save the file and test on your localhost.
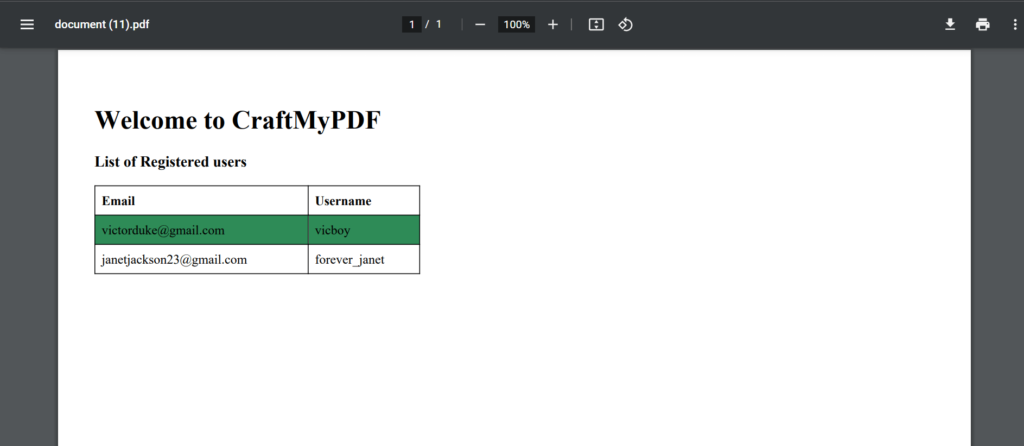
This code creates a PDF document with the following HTML and CSS layout.
1.3 TCPDF – One of the most used PHP libraries
TCPDF is a PHP library for generating PDF documents. It allows developers to easily create and manipulate PDF files using PHP code.
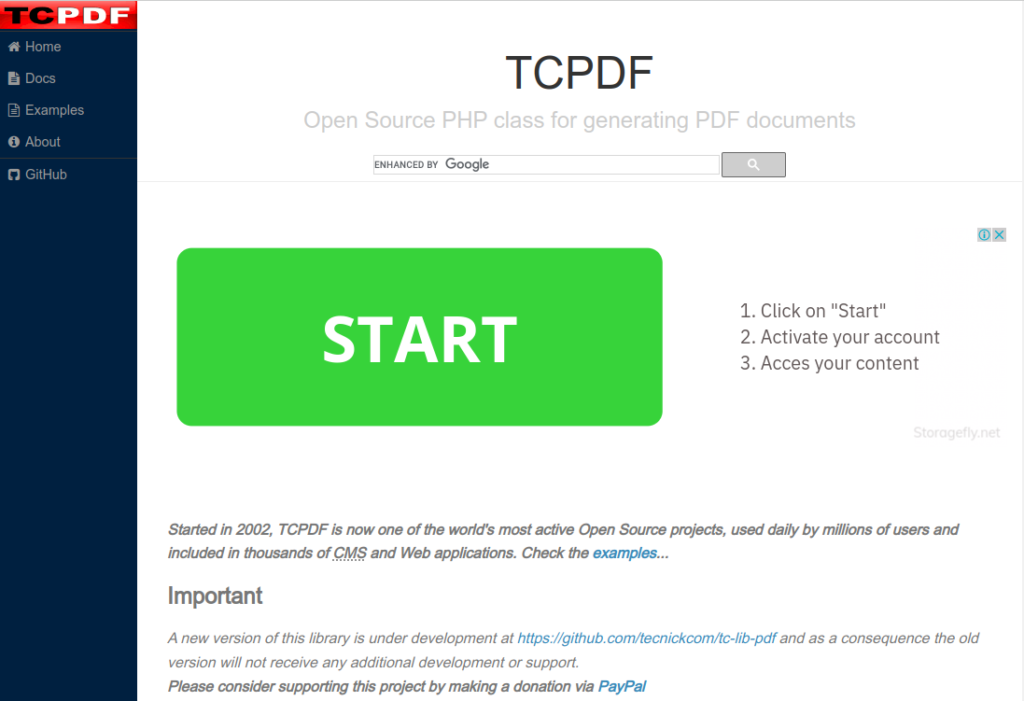
Built on PHP, it provides developers with a comprehensive set of features for creating complex and customizable PDFs programmatically.
It provides a wide range of features such as support for various fonts and Unicode characters, different page formats, header and footer customization, bookmarks, annotations, and digital signatures.
It also allows developers to add images, text, and vector graphics to the PDF document.
TCPDF has been used for various purposes, such as generating invoices, certificates, reports, and other types of documents. It is highly customizable and can be integrated with various PHP frameworks and applications.
In order for it to work, the following steps are necessary:
- Clone the repository.
- Create a file with the name ‘pdfScript.php’ (or any other desired name) within the cloned folder.
- Insert the provided code snippet into the created file
<?php
// Include the main TCPDF library (search for installation path).
require_once('tcpdf.php');
// create new PDF document
$pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
$pdf->SetCreator(PDF_CREATOR);
$pdf->SetAuthor('Nicole Asuni');
$pdf->SetTitle('PDF file using TCPDF');
// set default header data
$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE.' 006', PDF_HEADER_STRING);
// set header and footer fonts
$pdf->setHeaderFont(Array(PDF_FONT_NAME_MAIN, '', PDF_FONT_SIZE_MAIN));
$pdf->setFooterFont(Array(PDF_FONT_NAME_DATA, '', PDF_FONT_SIZE_DATA));
// set default monospaced font
$pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED);
// set margins
$pdf->SetMargins(20, 20, 20);
$pdf->SetHeaderMargin(PDF_MARGIN_HEADER);
$pdf->SetFooterMargin(PDF_MARGIN_FOOTER);
// set auto page breaks
$pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM);
// set image scale factor
$pdf->setImageScale(PDF_IMAGE_SCALE_RATIO);
// add a page
$pdf->AddPage();
$html = <<<EOD
<h1>TCPDF PHP Library is easy!</h1>
<p> My name is Ayeesha </p>
<i>This is an example of the TCPDF library.</i>
<p>I am using the default header and footer</p>
<p>Please check the source code documentation for more examples of using TCPDF.</p>
EOD;
// Print text using writeHTMLCell()
$pdf->writeHTMLCell(0, 0, '', '', $html, 0, 1, 0, true, '', true);
// $pdf->writeHTML($html, true, false, true, false, '');
// add a page
$pdf->AddPage();
$html = '<h4>Second page</h4>';
$pdf->writeHTML($html, true, false, true, false, '');
// reset pointer to the last page
$pdf->lastPage();
//Close and output PDF document
$pdf->Output('example.pdf', 'I');
?>
Save the file and test on your localhost
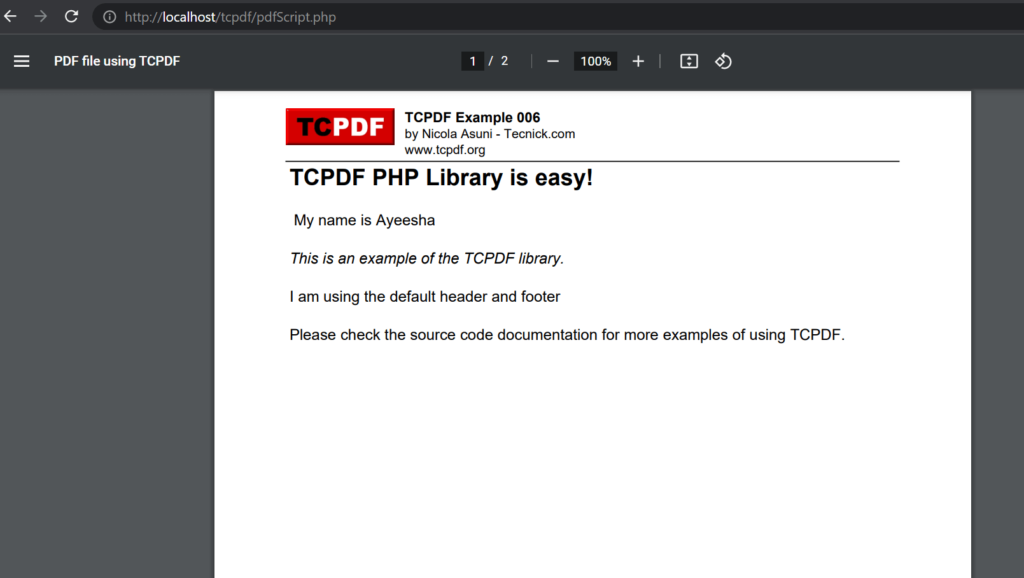
By using the TCPDF library’s default header and footer, a PDF document is created. Additional examples of how to implement the library can be located on their website.
1.4 wkhtmltopdf – WebKit rendering engine
wkhtmltopdf is a command-line tool that converts HTML documents to PDF.
It is based on the WebKit rendering engine, which allows it to accurately render HTML and CSS into a printable document format.
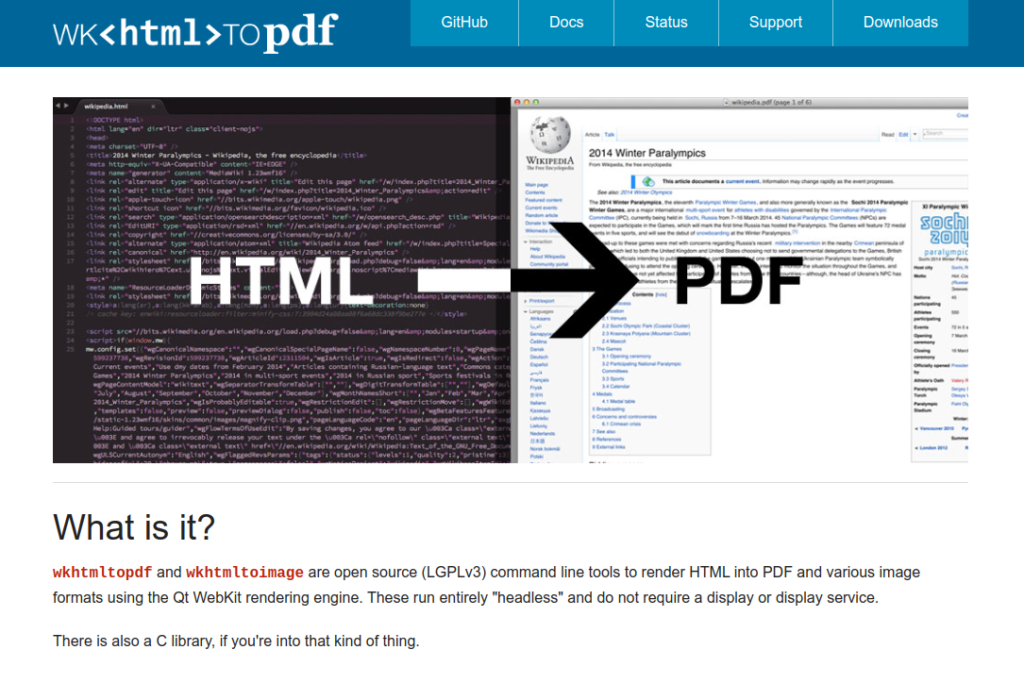
One downside of using wkhtmltopdf in PHP is its reliance on an external command-line tool. Since it’s not a native PHP library, integrating it into PHP applications requires executing shell commands, which can introduce complexity and potential security risks if not handled properly.
Here’s a step-by-step guide on how to generate PDF using wkhtmltopdf in PHP:
Step 1. Install wkhtmltopdf: First, you need to install wkhtmltopdf on your server. You can download it from the official website or use a package manager like apt or yum.
Step 2. Write your HTML content: Create the HTML content that you want to convert to PDF. This can be a dynamically generated HTML page or a static HTML file.
Step 3. Execute wkhtmltopdf command: You can execute the wkhtmltopdf command using PHP’s exec()
or shell_exec()
functions. Pass the path to the HTML file and the desired path for the output PDF file as arguments to wkhtmltopdf.
//
<?php
$htmlFilePath = "path/to/your/html/file.html";
$pdfFilePath = "path/to/output/pdf/file.pdf";
exec("wkhtmltopdf $htmlFilePath $pdfFilePath");
?>
//
Step 4. Handle errors: Check if the execution of wkhtmltopdf was successful and handle any errors accordingly.
//
<?php
$htmlFilePath = "path/to/your/html/file.html";
$pdfFilePath = "path/to/output/pdf/file.pdf";
$output = shell_exec("wkhtmltopdf $htmlFilePath $pdfFilePath 2>&1");
if (strpos($output, "Error") !== false) {
// Handle error
echo "Error occurred: $output";
} else {
// PDF generated successfully
echo "PDF generated successfully!";
}
?>
//
Serve the PDF: Once the PDF is generated, you can serve it to the user by providing a download link or displaying it directly in the browser.
That’s it! You’ve successfully generated a PDF using wkhtmltopdf in PHP. Make sure to handle any security considerations, such as sanitizing user input to prevent injection attacks when generating HTML content. Additionally, consider optimizing the HTML for print layout to ensure the best results in the generated PDF.
2. Comparison of FPDF, DOMPDF, TCPDF and wkhtmltopdf
When it comes to generating PDF documents dynamically in web applications, developers have several options to choose from. Among these, four popular libraries stand out: FPDF, DOMPDF, TCPDF, and wkhtmltopdf. Each of these libraries has its own strengths and weaknesses, catering to different use cases and preferences.
In this comparison, we’ll examine these libraries in terms of features, performance, ease of use, and community support to help developers make informed decisions when selecting the right tool for their PDF generation needs.
Feature | FPDF | DOMPDF | TCPDF | wkhtmltopdf |
---|---|---|---|---|
Language | PHP | PHP | PHP, written in PHP | Command line tool, can be used with any language |
Rendering Engine | Custom | HTML to PDF | Custom | WebKit (based on Qt) |
Ease of Use | Straightforward | Requires HTML/CSS knowledge | Moderate | Requires command line usage, HTML/CSS knowledge |
Performance | Efficient | Moderate | Efficient | Fast |
Features | Basic features, limited customization | Supports CSS2.1, limited CSS3 | Extensive features, customization | Supports CSS3, JavaScript, and more |
Community Support | Active | Active | Active | Active |
Comparison of all libraries:
- FPDF: FPDF is a PHP class that allows you to generate PDF files without using the PDFlib library. It offers basic features for creating PDFs and is relatively straightforward to use. However, customization options are limited compared to other libraries.
- DOMPDF: DOMPDF is a PHP library that converts HTML and CSS documents to PDF. It’s based on the DOMPDF library and supports CSS2.1 but has limited support for CSS3. While it’s easy to use for simple documents, complex layouts may require extra effort to achieve desired results.
- TCPDF: TCPDF is another PHP library for generating PDF documents. It provides extensive features and customization options, making it suitable for complex PDF generation tasks. TCPDF is efficient and actively maintained, with a large community contributing to its development.
- wkhtmltopdf: Unlike the PHP libraries mentioned above, wkhtmltopdf is a command-line tool that can be used with any programming language. It utilizes the WebKit rendering engine to convert HTML/CSS documents to PDF. This tool supports modern web technologies like CSS3 and JavaScript, making it ideal for generating PDFs from web pages.
These libraries cater to different needs, so the choice depends largely on the specific requirements of the project, such as performance considerations, the complexity of the PDF documents, and the development environment.
FPDF and TCPDF are optimal choices when prioritizing performance. DOMPDF, on the other hand, is lightweight and ideal for handling intricate documents, offering compatibility with HTML and CSS for customization.
Meanwhile, wkhtmltopdf excels in managing complex HTML, seamlessly integrating modern technologies such as CSS3 and Javascript.
3. Cloud-Based API Solution – CraftMyPDF’s PDF Generation API
CraftMyPDF offers a cloud-based PDF generation API that allows you to generate PDFs in PHP with its REST API. It comes with a web-based drag-and-drop PDF template designer for you to design PDF templates and generate eye-catching PDF documents using JSON data.
The followings are some of the features of CraftMyPDF:
- The ability to convert HTML to PDF
- Make use of reusable templates
- Drag-and-drop template design to create templates easily
- Compatibility with CSS, JavaScript, PHP, and Python
- Integration with various no-code platforms such as Zapier, n8n, Airtable, Bubble.io, and more
- Support for custom footer and header with page numbers and total pages
To generate a PDF document in PHP using CraftMyPDF, here’s what you need to do:
- Sign up/Login: Create an account on CraftMyPDF or log in if you already have an existing account. Don’t worry, you do not need to input your card details.
- Go to Manage Templates: After your registration is complete, you will be automatically directed to your dashboard. Go to Manage Templates which is located at the top of the page, on your “Manage Templates” page, select New PDF Template.
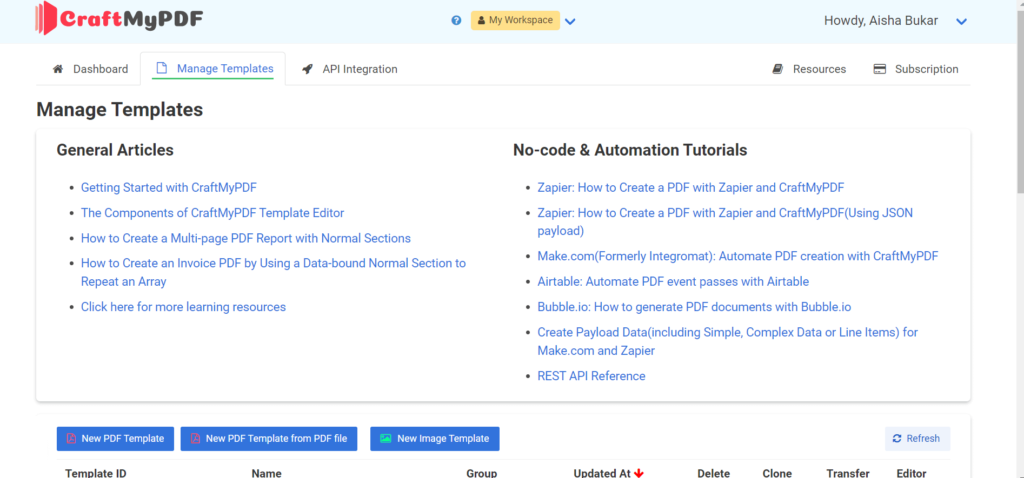
- Select New PDF Template: After selecting “New PDF Template”, you would be directed to a “Create a new template” page. You can decide to choose whatever template suits you best with the possibility of modifying it as you wish. I would be choosing the “Simple invoice” template.
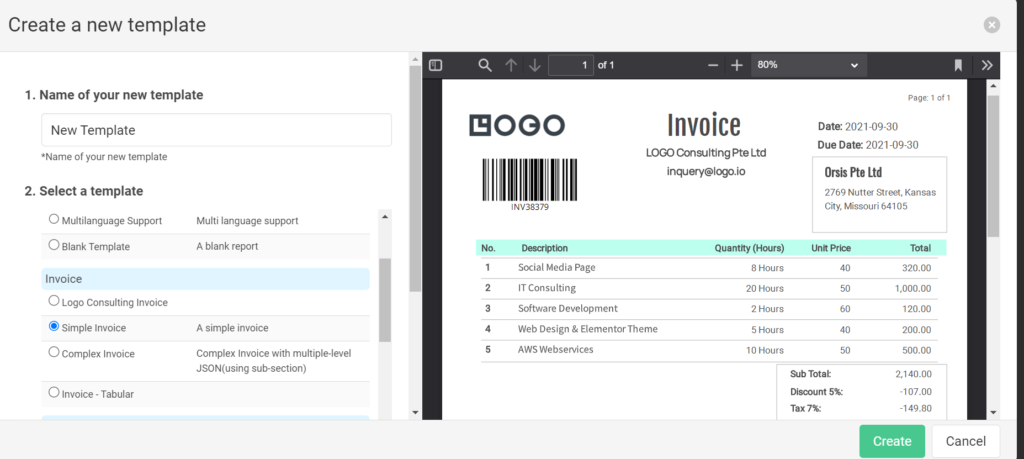
Create Template: Click on the Create template button. You will be redirected to your dashboard where you can view your template_id, edit, clone etc. You can also modify the name of your template (template_id) to whatever you like. Your new invoice template is ready to go!
Get API Key from “API Integration”: Now, back to your dashboard, click on the API Integration toolbar, and copy the default API key that has been generated for you. We would be using this API key to create our API endpoint so make sure it’s copied on your clipboard.
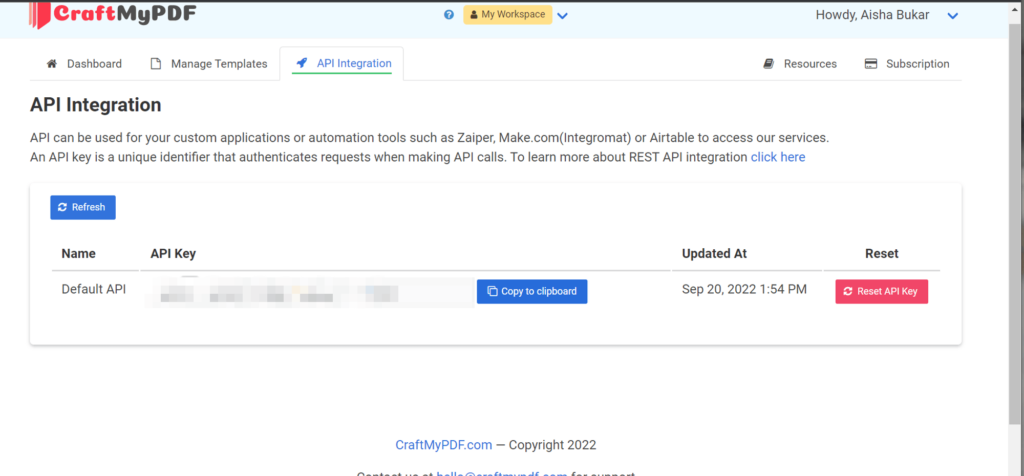
- Copy the following code snippet into your PHP script, we would be inserting some parameters like the template_id, API key, CraftMyPDF URL, and JSON data from our invoice.
<?php
function main() {
$api_key = "a4b8MTg3MzoxODczOnd2SEkwM1d3QUxnWUpw123";
$data = [
'invoice_number' => 'INV38379',
'date' => '2021-09-30',
'currency' => 'USD',
'due_date' => '2022-09-30',
'discount' => 5,
'tax' => 7,
'company_name' => 'LOGO Consulting Pte Ltd',
'email' => '[email protected]',
'client' => 'Orsis Pte Ltd',
'client_address' => '2769 Nutter Street,',
'client_address2' => 'Kansas City, Missouri',
'client_address3' => '64105',
'items' => [
[
'description' => 'AWS Webservices',
'quantity' => 10,
'unit_price' => 50,
'total' => 500
],
[
'description' => 'Web Design & Elementor Theme',
'quantity' => 5,
'unit_price' => 40,
'total' => 200
],
[
'description' => 'Software Development',
'quantity' => 2,
'unit_price' => 60,
'total' => 120
],
[
'description' => 'IT Consulting',
'quantity' => 20,
'unit_price' => 50,
'total' => 1000
],
[
'description' => 'Social Media Page',
'quantity' => 8,
'unit_price' => 40,
'total' => 320
]
],
'gross_total'=> 25055
]
;
$json_payload = [
"data" => json_encode($data),
"output_file" => "output.pdf",
"export_type" => "json",
"expiration" => 10,
"template_id" => "d1d77b2b2e24ae40"
];
$url = "https://api.craftmypdf.com/v1/create";
$headers = ["X-API-KEY: $api_key"];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($json_payload));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
}
main();
?>
Note: Replace the API key and the template_id with the correctly generated values for the script to work
This endpoint aims to produce a PDF document that incorporates both JSON data and a pre-defined template. We can test the script on our localhost to ensure it works. You should get an output similar to this.
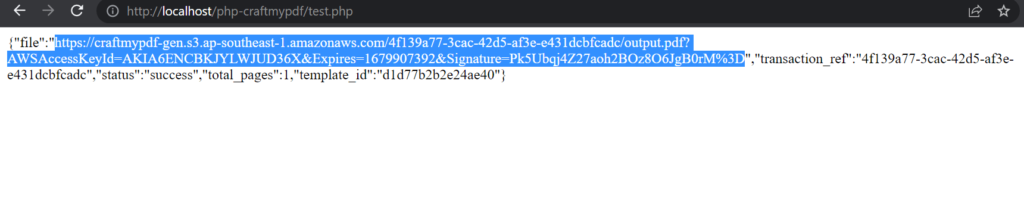
Copy the link to the PDF file and paste in your browser, the final output should look like this
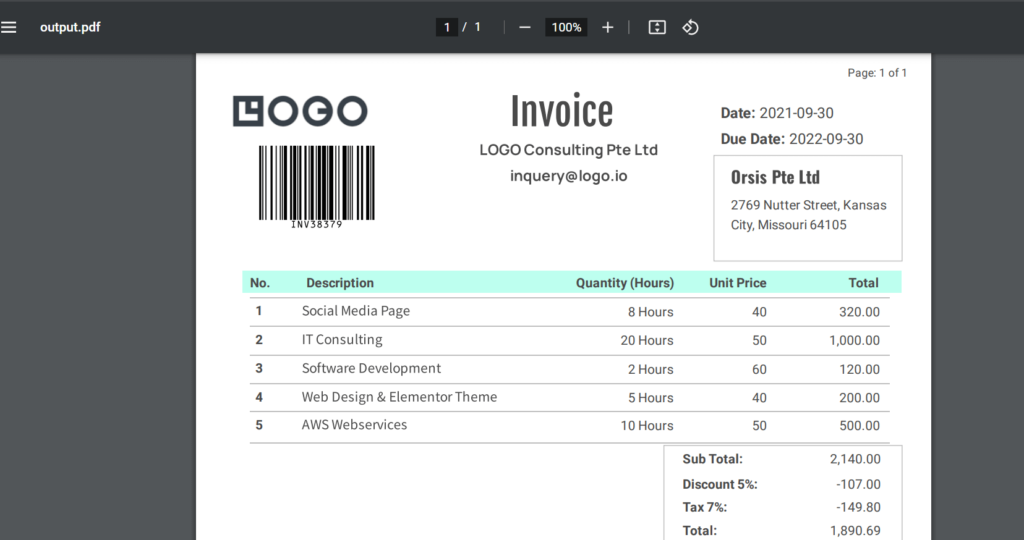
4. Test CraftmyPDF REST API with PostMan
We can also test the API on Postman, here’s what you need to do:
- Open Postman and create a new request by clicking on the “New” button.
- Select the HTTP method as
POST
. - In the request URL field, enter the API endpoint URL provided by the third-party API. In this case, the URL is
https://api.craftmypdf.com/v1/create
. - In the request HEADERS section, add a new header with the key
X-API-KEY
and the value of your API key (a4b8MTg3MzoxODczOnd2SEkwM1d3QUxnx1234). This header is required for authentication. - In the request body section, select the
raw
format and chooseJSON
as the data type. - Paste the following JSON payload in the request body field:
{
"data": {
"invoice_number": "INV38379",
"date": "2021-09-30",
"due_date": "2021-10-30",
"currency": "USD",
"tax": 7,
"company_name": "LOGO consulting",
"email": "[email protected]",
"client": "OpenAI Ltd",
"client_address": "20 New York, NJ",
"client_address2": "Washington, DC",
"client_address3": "Akansas",
"items": [
{
"description": "AWS Web services",
"quantity": 10,
"unit_price": 50,
"total": 100
},
{
"description": "Web Design & Elementor Theme",
"quantity": 5,
"unit_price": 40,
"total": 200
},
{
"description": "Software Development",
"quantity": 2,
"unit_price": 60,
"total": 120
},
{
"description": "IT Consulting",
"quantity": 20,
"unit_price": 50,
"total": 1000
},
{
"description": "Social Media Page",
"quantity": 8,
"unit_price": 40,
"total": 320
}
],
"total_amount": 82542.56
},
"load_data_from": null,
"template_id": "d1d77b2b2e24ae40",
"version": 8,
"export_type": "json",
"expiration": 60,
"output_file": "output.pdf",
"is_cmyk": false,
"image_resample_res": 600,
"direct_download": 0,
"cloud_storage": 1
}
This is the same payload that is being used in the PHP code.
7. Click on the “Send” button to send the request. If everything goes well, you should receive a response with the download URL for the generated PDF file similar to the screenshot below.
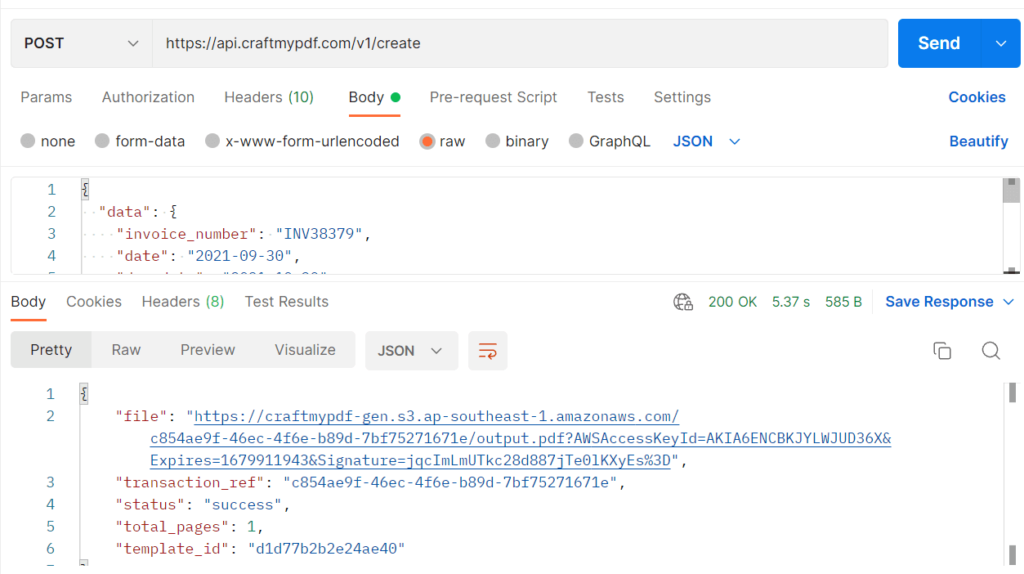
With the URL, you should be able to generate a PDF similar to the image below.
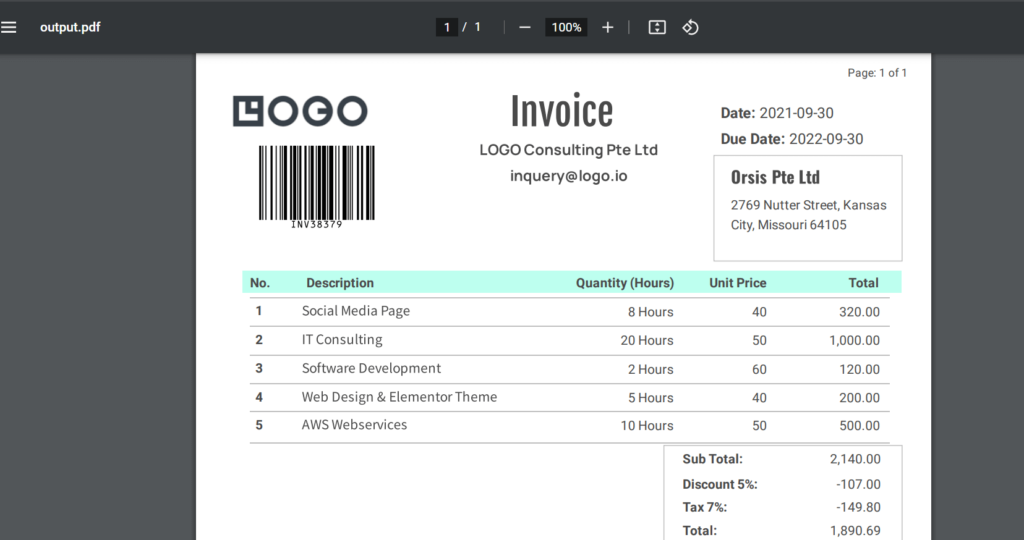
5. Benefits of CraftMyPDF
CraftMyPDF offers a drag-and-drop editor that gives you the ability to generate high-quality PDFs quickly and easily, without the need for complex coding or design skills. The following are some of the features:
i. Integration with various no-code platforms such as Zapier, n8n, Airtable, Bubble.io, and more.
ii. Access to a diverse range of quality fonts. Our font selection supports over 25 languages including Latin, Greek, Arabic, Japanese, Chinese, Vietnamese, Korean, Hebrew, Arabic, Tamil, and more.
iii. Our PDF editor can be seamlessly integrated into your application.
iv. Expressions and formatting for datetime, currency, and custom formats are fully supported by our PDF template editor. Additionally, these expressions can be utilized to incorporate headers and footers into your PDF document.
v. It allows you to create fillable PDF templates smoothly with our drag-and-drop editor.
6. Conclusion
There are several ways to generate PDF documents using PHP, including libraries such as FPDF, Dompdf, TCPDF and wkhtmltopdf, as well as using a cloud-based service like CraftMyPDF.
With the ability to create customized PDFs, incorporating different styles and templates, you can effectively communicate your ideas to your target audience. So, whether you’re a developer who needs to create PDFs regularly, there are plenty of options available to help you get the job done.
To create and design PDF templates using CraftMyPDF’s drag-and-drop editor, sign up now for free and start experimenting with our PDF generation API.